일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | ||||||
2 | 3 | 4 | 5 | 6 | 7 | 8 |
9 | 10 | 11 | 12 | 13 | 14 | 15 |
16 | 17 | 18 | 19 | 20 | 21 | 22 |
23 | 24 | 25 | 26 | 27 | 28 |
- 315. Count of Smaller Numbers After Self
- LeetCode
- Convert Sorted List to Binary Search Tree
- 밴픽
- Python
- 운영체제
- Substring with Concatenation of All Words
- concurrency
- Python Code
- shiba
- 109. Convert Sorted List to Binary Search Tree
- 43. Multiply Strings
- kaggle
- 30. Substring with Concatenation of All Words
- data science
- attribute
- iterator
- Decorator
- 프로그래머스
- DWG
- 컴퓨터의 구조
- Python Implementation
- 파이썬
- Protocol
- Class
- t1
- Regular Expression
- 715. Range Module
- 시바견
- Generator
- Today
- Total
목록분류 전체보기 (407)
Scribbling
First solution is using regular expression. Below is some useful patterns. Integer: ^[+-]?\d+$ Flaot: ^[+-]?((\d+(\.\d*)?)|(\.\d+))$ Sicnetific notation: ^[+-]?((\d+(\.\d*)?)|(\.\d+))[eE][+-]?\d+$ class Solution: def isNumber(self, s: str) -> bool: import re pat = re.compile('(^[+-]?((\d+(\.\d*)?)|(\.\d+))$)|(^[+-]?((\d+(\.\d*)?)|(\.\d+))[eE][+-]?\d+$)') return re.match(pat, s) Second solution i..
Refer to the website below for the detailed instructions. https://www.w3schools.com/python/python_ref_string.asp Python String Methods W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more. www.w3schools.com 1. len(str) - returns the length of the string ..
A solution using sliding window. class Solution: def findSubstring(self, s: str, words: List[str]) -> List[int]: from collections import Counter ret = [] wordSet = set(words) n, m = len(words), len(words[0]) for i in range(m): wordCounter = Counter(words) k = j = i while j + m = n * m: if s[k:k+m] in wordSet: wordCounter[s[k:k+m]] += 1 k += m word = s[j:j+m] if word in wordSet: wordCounter[word]..
class Solution: def removeElement(self, nums: List[int], val: int) -> int: length = len(nums) i = 0 while i
First thing to try is iterating nums1, nums2, nums3 and looking for the corresponding element in nums4. This will result in O(N**3) time complexity, which raises TLE. class Solution: def fourSumCount(self, nums1: List[int], nums2: List[int], nums3: List[int], nums4: List[int]) -> int: from collections import Counter c = Counter(nums4) ret = 0 for n1 in nums1: for n2 in nums2: for n3 in nums3: re..
Idea: Any characters that have frequencies less than k work as a breakpoints, meaning that the substring we're looking for can't include that particular character. So, recursively look for substrings while excluding those characters. Time complexity is O(N). from collections import Counter class Solution: def longestSubstring(self, s: str, k: int) -> int: return self.helper(s, k) def helper(self..
Refer to the below post for Knuth Shuffle. https://focalpoint.tistory.com/253 Shuffle Algorithm: Knuth Shuffle This post is about Knuth Shuffle, a shuffle algorithm. - This algorithm allows random shuffle, giving all permuations of array equal likelihood. - No need for additional arrays, so memory complexity.. focalpoint.tistory.com class Solution: def __init__(self, nums: List[int]): self.nums ..
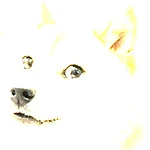
This post is about Knuth Shuffle, a shuffle algorithm. - This algorithm allows random shuffle, giving all permuations of array equal likelihood. - No need for additional arrays, so memory complexity is O(1). - Time complexity is O(N) Code is pretty simple as below. To give all permuations of a particular array equal likelihood, all the number should have the same probability to be placed in a ce..
Coming up with O(1) memory solution was very difficult for me. I refered to this post's last solution. https://leetcode.com/problems/kth-smallest-element-in-a-sorted-matrix/discuss/1322101/C%2B%2BJavaPython-MaxHeap-MinHeap-Binary-Search-Picture-Explain-Clean-and-Concise [C++/Java/Python] MaxHeap, MinHeap, Binary Search - Picture Explain - Clean & Concise - LeetCode Discuss Level up your coding s..
Making a new list is an easy solution to implement with recursion. class NestedIterator: def __init__(self, nestedList: [NestedInteger]): self.res = [] self._flattener(nestedList) self.ptr = 0 def _flattener(self, nestedList): for nl in nestedList: if nl.isInteger(): self.res.append(nl.getInteger()) else: self._flattener(nl.getList()) def next(self) -> int: ret = self.res[self.ptr] self.ptr += 1..