일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | 6 | |
7 | 8 | 9 | 10 | 11 | 12 | 13 |
14 | 15 | 16 | 17 | 18 | 19 | 20 |
21 | 22 | 23 | 24 | 25 | 26 | 27 |
28 | 29 | 30 |
- Protocol
- Python Implementation
- Generator
- 109. Convert Sorted List to Binary Search Tree
- 715. Range Module
- attribute
- Regular Expression
- 컴퓨터의 구조
- 파이썬
- Class
- 시바견
- kaggle
- 프로그래머스
- data science
- DWG
- t1
- 밴픽
- 315. Count of Smaller Numbers After Self
- Substring with Concatenation of All Words
- Convert Sorted List to Binary Search Tree
- Decorator
- 43. Multiply Strings
- LeetCode
- Python Code
- shiba
- 운영체제
- concurrency
- iterator
- Python
- 30. Substring with Concatenation of All Words
- Today
- Total
목록LeetCode (205)
Scribbling
How does one can find the number of pairs in an array that satisfies the below condition? : lower
A dynamic programming approach: Let dp[i, j] be the total number of cases of target[i] corresponding to jth position dp[i, j] = dp[i][j-1] + dp[i-1][j-1] * counter[j, target[i]] 1) Bottom-up import string from functools import lru_cache class Solution: def numWays(self, words: List[str], target: str) -> int: N = len(words[0]) freqs = [{c: 0 for c in string.ascii_lowercase} for _ in range(N)] for..
class TrieNode { public boolean isWord = false; public TrieNode[] children = new TrieNode[26]; } class Trie { public TrieNode root; public Trie() { root = new TrieNode(); } public void insert(String word) { TrieNode cur = root; for (int i=0; i
Greedy solution with heap & deque - append character with 'max frequency' currently - put the appended character to a deque for cool-down from collections import Counter, deque import heapq class Solution: def rearrangeString(self, s: str, k: int) -> str: if k = k: freq, char = q.popleft() if freq >= 1: heapq.heappush(h, (-freq, char)) return ''.join(ret) if len(ret) == len(s) else ""
Fenwick Tree (or Binary Indexed Tree) is very efficient in dealing with range queries, especially when elements get updated occasionally. For how it works, refer to the below video. He elaborates on the principle of the tree in a very clean way. https://www.youtube.com/watch?v=CWDQJGaN1gY&t=160s&ab_channel=TusharRoy-CodingMadeSimple Below is the python implementation of it. class Fenwick: """ Py..
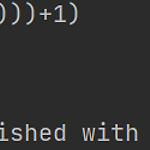
Video I recommend: https://www.youtube.com/watch?v=SToUyjAsaFk In this post, I will cover "Recursive Descent Parsing". Details about it are well elaborated in the above video. This post is to summarize core concepts and implement the algorithm with an actual code. Goal: Implement a parser that parse an arithmetic experssion. - ( 3 + 5) * -2 Elements: Numbers Infix Operators (+, -, *, /) Prefix O..
Python does not provide standard library for ordered set or ordered dictionary. However, there's an Apache2 licensed sorted collections library "SortedContainer". You can install the library with the following cmd. pip install sortedcontainers https://www.geeksforgeeks.org/python-sorted-containers-an-introduction/#:~:text=Sorted%20set%20is%20a%20sorted,must%20be%20hashable%20and%20comparable. Py..
I found this problem is pretty similar to 'Russian Doll' prob (https://leetcode.com/problems/russian-doll-envelopes/). Thought process for this problem is: 0> Sort the properties. 1> Let's just assume that attack strictly increases (e.g. 3, 4, 5, ...): then what we should do is to count how many defense values have larger values on their right side. And that's exactly what monotonicly desc stack..
크게 두 단계로 풀 수 있다. 1) 알파벳 조합이 동일한 집합끼리 나눈다. 2) 각 집합 내에서 단어를 연결하고, 소 집합의 갯수를 구한다. This prob can be solved within two steps. 1) Group words by alphabet combinations 2) Connect words in each group and then get the number of small groups Code is quite straightforward, so I guess there's no need to explain more. Time complexity would be (O(N**2*k)), where N is the number of words and k is length of a w..
For a given recipe, we need to check whether all the necessary elements can be supplied. If an element belongs to supplies, it's a piece of cake. Now the question is to deal with elements that belong to recipes. We can think of recipes as a graph with cycles. Plus, if there's a cycle among certain recipes, they can never be cooked. class Solution: def findAllRecipes(self, recipes: List[str], ing..