일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | ||||||
2 | 3 | 4 | 5 | 6 | 7 | 8 |
9 | 10 | 11 | 12 | 13 | 14 | 15 |
16 | 17 | 18 | 19 | 20 | 21 | 22 |
23 | 24 | 25 | 26 | 27 | 28 |
- 315. Count of Smaller Numbers After Self
- 프로그래머스
- Python Implementation
- 시바견
- Python Code
- Substring with Concatenation of All Words
- Regular Expression
- 운영체제
- Protocol
- data science
- 43. Multiply Strings
- Convert Sorted List to Binary Search Tree
- concurrency
- Generator
- 30. Substring with Concatenation of All Words
- shiba
- Decorator
- Class
- t1
- kaggle
- 109. Convert Sorted List to Binary Search Tree
- iterator
- 밴픽
- attribute
- 컴퓨터의 구조
- LeetCode
- 715. Range Module
- 파이썬
- Python
- DWG
- Today
- Total
목록분류 전체보기 (407)
Scribbling
#include #include using namespace std; class Solution { public: vector twoSum(vector& nums, int target) { vector res; unordered_map hash; for (int i = 0; i < nums.size(); i++) { int num = target - nums[i]; // found if (hash.find(num) != hash.end()) { res.push_back(hash[num]); res.push_back(i); return res; } hash[nums[i]] = i; } return res; } }; int main() { vector vec{ 2, 7, 11, 15 }; Solution()..
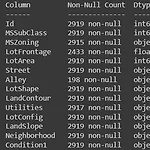
Data Science 101 1. Data Type and Null Check all_df.info() 1.1. Nullity #missing data total = all_df.isnull().sum().sort_values(ascending=False) percent = (all_df.isnull().sum()/all_df.isnull().count()).sort_values(ascending=False) missing_data = pd.concat([total, percent], axis=1, keys=['Total', 'Percent']) missing_data = missing_data.drop(['SalePrice'], axis=0) missing_data.head(20) 1.2. Featu..
class Queue: def __init__(self, size): self.size = size self.q = [0] * size self.cnt = 0 self.head, self.tail = 0, 0 def append(self, val): if self.cnt == self.size: print('Full Queue Error') return False self.cnt += 1 self.q[self.tail] = val self.tail += 1 if self.tail == self.size: self.tail = 0 return True def popleft(self): if self.cnt == 0: print('Empty Queue Error') return False self.cnt -..
1. Appending Rows to empty DataFrame tt = pd.DataFrame() tt = tt.append({'Date': date, 'Location': country, 'Sadness': arr[0], 'Anger': arr[1], 'Joy': arr[2], 'Optimism': arr[3]}, ignore_index=True) 2. Row Display Option pd.set_option('display.max_columns', 100)
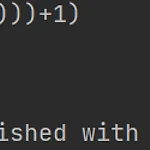
Video I recommend: https://www.youtube.com/watch?v=SToUyjAsaFk In this post, I will cover "Recursive Descent Parsing". Details about it are well elaborated in the above video. This post is to summarize core concepts and implement the algorithm with an actual code. Goal: Implement a parser that parse an arithmetic experssion. - ( 3 + 5) * -2 Elements: Numbers Infix Operators (+, -, *, /) Prefix O..
Rabin-Karp Algorithm is an efficient algorithm to search a string pattern in a given sentence. The core of the idea is to use hash function to compare strings. Time complexity is O(n), where n is length of the string. However, this is true only when there is no hash collision. Time complexity is O(n * m) if hash function is poorly selected (producing many hash collisions), where m is length of t..
Python does not provide standard library for ordered set or ordered dictionary. However, there's an Apache2 licensed sorted collections library "SortedContainer". You can install the library with the following cmd. pip install sortedcontainers https://www.geeksforgeeks.org/python-sorted-containers-an-introduction/#:~:text=Sorted%20set%20is%20a%20sorted,must%20be%20hashable%20and%20comparable. Py..
I found this problem is pretty similar to 'Russian Doll' prob (https://leetcode.com/problems/russian-doll-envelopes/). Thought process for this problem is: 0> Sort the properties. 1> Let's just assume that attack strictly increases (e.g. 3, 4, 5, ...): then what we should do is to count how many defense values have larger values on their right side. And that's exactly what monotonicly desc stack..
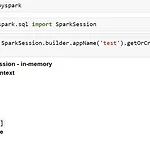
In this post, I will review basics of Spark, especially pySpark. Spark is a framework for handling big data and has a great strength in distributed system with multiple nodes. To install pyspark, simply 'pip install pyspark'. For demonstration, I will use 'heart.csv' dataset from https://www.kaggle.com/datasets/johnsmith88/heart-disease-dataset. Now, let's get down to the code. In pyspark, we ca..
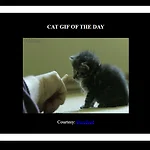
From installation to basic concepts, I recommend the video below. https://www.youtube.com/watch?v=hWPv9LMlme8 Below is an official tutorial for docker beginners. This post is a short step-by-step review of it. https://docker-curriculum.com/#our-first-image A Docker Tutorial for Beginners Learn to build and deploy your distributed applications easily to the cloud with Docker docker-curriculum.com..