일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | ||||||
2 | 3 | 4 | 5 | 6 | 7 | 8 |
9 | 10 | 11 | 12 | 13 | 14 | 15 |
16 | 17 | 18 | 19 | 20 | 21 | 22 |
23 | 24 | 25 | 26 | 27 | 28 |
- concurrency
- kaggle
- 운영체제
- DWG
- Decorator
- Class
- Python Implementation
- Python Code
- 프로그래머스
- 밴픽
- Generator
- shiba
- Convert Sorted List to Binary Search Tree
- 715. Range Module
- 시바견
- 30. Substring with Concatenation of All Words
- t1
- 315. Count of Smaller Numbers After Self
- iterator
- 컴퓨터의 구조
- Protocol
- 파이썬
- Substring with Concatenation of All Words
- data science
- LeetCode
- Regular Expression
- Python
- 109. Convert Sorted List to Binary Search Tree
- 43. Multiply Strings
- attribute
- Today
- Total
목록분류 전체보기 (407)
Scribbling
To learn sequence protocol in Python, we create a custom vector class. from array import array import math import reprlib class Vector: typecode = 'd' def __init__(self, components): self._components = array(self.typecode, components) def __iter__(self): return iter(self._components) def __repr__(self): components = reprlib.repr(self._components) components = components[components.find('['):-1] ..
What is a "Pythonic Object"? An object with many different magic methods seems to be more pythonic. However, it doesn't necessarily refer to a class with a lot of magic methods. That is, we don't have to implement all the magic methods in every class. Instead, implement them if only needed, because 'being simple' is pythonic. Below is Vector2D class example. from array import array import math c..
ID operator returns the object's memory address. We use it to compare objects' identities. morgan = {'name': 'hoesu', 'age':29} hoesu = morgan print(hoesu is morgan) print(id(hoesu), id(morgan)) "==" operator calls __eq__() method in dict class and checks whether the objects have the same value or not. morgan = {'name': 'hoesu', 'age':29} anonymous = {'name': 'hoesu', 'age':29} print(morgan == a..
Decorator is a callable that takes another function as its parameter. Decorator is executed during 'import time'. The key idea about closure you must understand is that it can access to 'nonlocal' variables outside the function. Take a look at the below exmaple. def make_averager(): series = [] def averager(new_val): series.append(new_val) return sum(series) / len(series) return averager avg = m..
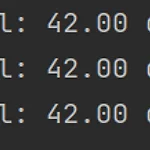
Some traditional design patterns can be simplified by making good use of first-order functions in python. Let's see the below example. 1. Goal: Calculate total price and discount for each order 2. There are three discount strategies; - fidelitypromo: if the customer's fidelity >= 1000, then 5% of the total - bulkitempromo: if q >= 20 for an item, then 10% of the item - largeorderpromo: if type >..
First thing I came out with was to use a trie data structure, as it helps us deal with characters efficiently. class StreamChecker: def __init__(self, words: List[str]): self.trie = {} for word in words: node = self.trie for char in word: if char not in node: node[char] = {} node = node[char] node['#'] = '#' self.candidates = [] def query(self, letter: str) -> bool: self.candidates.append(self.t..
The US oil industry doesn’t appear to be in rush to increase production despite the surging prices. The oil industry is restraining growth and it is largely because of capital discipline from Wall Street, according to a Federal Reserve Bank of Dallas survey. A few years ago, the industry invested fortune in production growth. However, it resulted in keeping oil prices low which led hundreds of o..
1. User defined Callable Object User defined callable object can be defined as below. Magic method __call__ should be implemented. import random class BingoCage: def __init__(self, items): self.__items = list(items) random.shuffle(self.__items) def __pick(self): try: return self.__items.pop() except IndexError: raise LookupError('pick from empty BingoCage') def __call__(self): return self.__pick..
Python memoryview function returns memory view objects. So why use it? Memory view is a safe way to expose 'buffer protocol' in python. Buffer protocol allows an object to expose its inner data without copying. As buffer protocol is only available at the C-API level, memoryview is present to expose the same protocol at Python level. Example1: how memoryview works arr = bytearray('XYZ', 'utf-8') ..
1. Using heaps with lazy removal Below code uses 'MyHeap' data structure, a heap with lazy removal. Lazy removal here means, if we have to remove an element, we handle it by using another heap as a trash can. import heapq class MyHeap: def __init__(self, type='min'): self.heap = [] self.removals = [] self.sign = 1 if type == 'min' else -1 def insert(self, elem): heapq.heappush(self.heap, self.si..